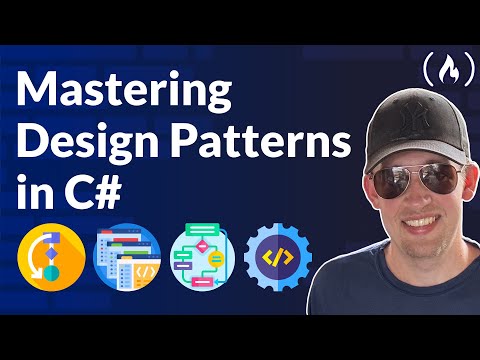
Curriculum for the course Master Design Patterns & SOLID Principles in C# - Full OOP Course for Beginners
In this comprehensive and beginner-friendly course, you will learn all of the tools that you need to become an advanced OOP programmer, writing clean and maintainable software. What you will learn: - Fundamental OOP concepts, such as inheritance, composition, encapsulation, abstraction, composition vs inheritance, fragile base class problem. - Unified Modeling Language (UML) to model your classes and objects, and the relationships between them in a graphical way. - All five SOLID Principles. - All 23 "Gang of Four" software design patterns. 💻 Github repo: https://github.com/DoableDanny/Design-Patterns-in-C-Sharp ✏️ Course created by Dan Adams. Check out his channel: https://www.youtube.com/@doabledanny?sub_confirmation=1 A book and cheatsheet for this course are also available: 🔗 eBook and design patterns cheatsheet PDF: https://doabledanny.gumroad.com/l/ennyj 🔗 Amazon Kindle eBook & physical book: https://www.amazon.com/Mastering-Design-Patterns-Beginner-Friendly-Principles/dp/B0DBZGQZMZ ⭐️ Contents ⭐️ (0:00:00) Intro (0:00:33) Course contents (0:01:34) Gang of Four design patterns (0:02:39) What are design patterns & why learn them? (0:05:38) Course prerequisites (0:06:57) About me (0:07:32) Book version (0:08:19) Code repo (0:08:49) Setup (0:12:19) OOP concepts intro (0:12:42) Encapsulation - OOP (0:25:48) Abstraction - OOP (0:30:52) Inheritance - OOP (0:36:40) Polymorphism - OOP (0:45:04) Coupling - OOP (0:55:17) Composition - OOP (0:58:11) Composition vs inheritance - OOP (1:01:00) Fragile base class problem - OOP (1:05:24) UML (1:14:01) SOLID intro (1:15:01) S - SOLID (1:21:26) O - SOLID (1:32:20) L - SOLID (1:45:20) I - SOLID (1:54:10) D - SOLID (2:04:56) Design patterns intro (2:05:35) Behavioural design patterns (2:07:37) Memento pattern - behavioural (2:33:40) State pattern - behavioural (3:00:27) Strategy pattern - behavioural (3:26:47) Iterator pattern - behavioural (3:46:09) Command pattern - behavioural (4:24:17) Template method pattern - behavioural (4:56:50) Observer pattern - behavioural (5:31:20) Mediator pattern - behavioural (6:10:19) Chain of responsibility pattern - behavioural (6:42:55) Visitor pattern - behavioural (7:06:29) Interpreter pattern - behavioural (7:38:53) Structural design patterns intro (7:40:32) Composite pattern - structural (7:56:09) Adapter pattern - structural (8:13:26) Bridge pattern - structural (8:33:16) Proxy pattern - structural (8:51:33) Flyweight pattern - structural (9:15:25) Facade pattern - structural (9:27:13) Decorator pattern - structural (9:55:16) Creational design patterns intro (9:58:50) Prototype pattern - creational (10:19:13) Singleton pattern - creational (10:37:44) Factory method pattern - creational (10:55:03) Abstract factory pattern - creational (11:12:26) Builder pattern - creational (11:46:29) Course conclusionWatch Online Full Course: Master Design Patterns & SOLID Principles in C# - Full OOP Course for Beginners
Click Here to watch on Youtube: Master Design Patterns & SOLID Principles in C# - Full OOP Course for Beginners
This video is first published on youtube via freecodecamp. If Video does not appear here, you can watch this on Youtube always.
Udemy Master Design Patterns & SOLID Principles in C# - Full OOP Course for Beginners courses free download, Plurasight Master Design Patterns & SOLID Principles in C# - Full OOP Course for Beginners courses free download, Linda Master Design Patterns & SOLID Principles in C# - Full OOP Course for Beginners courses free download, Coursera Master Design Patterns & SOLID Principles in C# - Full OOP Course for Beginners course download free, Brad Hussey udemy course free, free programming full course download, full course with project files, Download full project free, College major project download, CS major project idea, EC major project idea, clone projects download free
Comments
Post a Comment